The process is:
first get a list of stocks from a screener
look at the chart for each stock and sort it to a category
(only if that stock hasn't previously been sorted)
some categories will be for the record only
others will be for watching
periodically or at intervals look at all the stocks on each for watching list
make a new record for each stock if the chart is interesting
Tuesday, February 28, 2017
sorting from a sorted list
function sortthisrecord() {
var thenewrecord = document.getElementById("sns_theinput").value
var thesortedlist = document.getElementById("sns_thesortedlist")
var thesortedlistarray = thesortedlist.innerHTML.split(";")
thesortedlistarray.push(thenewrecord)
thesortedlist.innerHTML = thesortedlistarray.join(";")}
var thenewrecord = document.getElementById("sns_theinput").value
var thesortedlist = document.getElementById("sns_thesortedlist")
var thesortedlistarray = thesortedlist.innerHTML.split(";")
thesortedlistarray.push(thenewrecord)
thesortedlist.innerHTML = thesortedlistarray.join(";")}
sort a sorted list
function sortasortedlist(listname) {
document.getElementById("sns_thislist").innerHTML = getthislist(listname)
loadfirstrecord()}
function loadfirstrecord() {
loadarecordfromthelist(0)}
function loadarecordfromthelist(therecordnumber) {
var thelist = document.getElementById("sns_thislist")
var thelistarray = thelist.innerHTML.split(";")
var thisrecord = thelistarray[therecordnumber]
var thisrecordarray = thisrecord.split(",")
var firstrecord = thisrecordarray[0]
var thesymbol = firstrecord.split("/")[firstrecord.split("/").length - 1].split("c"
http://stackoverflow.com/questions/1027224/how-can-i-test-if-a-letter-in-a-string-is-uppercase-or-lowercase-using-javascrip
function thesymbol(therecord) {
var therecordparts = therecord.split("/")
var thelastrecordpart = therecordparts[threcordparts.length - 1]
var thepositionofthelettertocheck = 0
var theletter = thelastrecordpart[thepositionofthelettertocheck]
var thesymbol = ""
while (iscapq(theletter) === "yes") {
thesymbol = thesymbol + theletter
thepositionofthelettertocheck = thepositionofthelettertocheck + 1
theletter = thelastrecordpart[thepositionofthelettertocheck]}
return thesymbol}
document.getElementById("sns_thislist").innerHTML = getthislist(listname)
loadfirstrecord()}
function loadfirstrecord() {
loadarecordfromthelist(0)}
function loadarecordfromthelist(therecordnumber) {
var thelist = document.getElementById("sns_thislist")
var thelistarray = thelist.innerHTML.split(";")
var thisrecord = thelistarray[therecordnumber]
var thisrecordarray = thisrecord.split(",")
var firstrecord = thisrecordarray[0]
var thesymbol = firstrecord.split("/")[firstrecord.split("/").length - 1].split("c"
http://stackoverflow.com/questions/1027224/how-can-i-test-if-a-letter-in-a-string-is-uppercase-or-lowercase-using-javascrip
function thesymbol(therecord) {
var therecordparts = therecord.split("/")
var thelastrecordpart = therecordparts[threcordparts.length - 1]
var thepositionofthelettertocheck = 0
var theletter = thelastrecordpart[thepositionofthelettertocheck]
var thesymbol = ""
while (iscapq(theletter) === "yes") {
thesymbol = thesymbol + theletter
thepositionofthelettertocheck = thepositionofthelettertocheck + 1
theletter = thelastrecordpart[thepositionofthelettertocheck]}
return thesymbol}
sorting from an unsorted list
function sortastock() {
document.getElementById("sns_therecord").style.display = "none"
var theunsortedlist = document.getElementById("sns_theunsortedlist")
var thesortedlist = document.getElementById("sns_thesortedlist")
var thesorter = document.getElementById("sns_sorter")
var updaterstate = document.getElementById("sns_updaterstate")
var theflink = document.getElementById("sns_theflink")
var themlink = document.getElementById("sns_themlink")
var thesymbol = findthestock(theunsortedlist,thesortedlist)
thesorter.src = updatethesorter(updaterstate.innerHTML,thesymbol)
theflink.href = "http://elite.finviz.com/quote.ashx?t=" + thesymbol
themlink.href = "http://www.marketwatch.com/investing/stock/" + thesymbol}
function sortthisstock() {
var thisstock = document.getElementById("sns_input").value
install the sorted list
function installthesortedlist() {
var thelistsource = document.getElementById("sns_input").value
document.getElementById("sns_sortedlistrepository").innerHTML = thelistsource}
var thelistsource = document.getElementById("sns_input").value
document.getElementById("sns_sortedlistrepository").innerHTML = thelistsource}
install the unsorted list
function installtheunsortedlist() {
var thelistsource = document.getElementById("sns_input").value
document.getElementById("sns_unsortedlistrepository").innerHTML = thelistsource}
var thelistsource = document.getElementById("sns_input").value
document.getElementById("sns_unsortedlistrepository").innerHTML = thelistsource}
sorter functions index
setting up the sorter a new approach!
sorting from an unsorted list the application
sorting from a sorted list build the application
viewing the details of a record
the ui
Saturday, February 25, 2017
thinking about the sorter
Thinking that the sorter ...
<textarea id="msns20170225sa1747i"></textarea>
<a href="javascript:msns20170225sa1747('get unsorted list')">
create new list after pasting in csv file</a>
<a href="javascript:msns20170225sa1747('sort to list')">
paste in three record urls and a list name like this: url,url,url,list name to sort this stock to a list</a>
<div id="msns20170225sa1747unsortedlist"></div>
<div id="msns20170225sa1747sortedlist"></div>
<div id="msns20170225sa1747listname"></div>
<div id="msns20170225sa1747currentposition"></div>
<textarea id="msns20170225sa1747i"></textarea>
<a href="javascript:msns20170225sa1747('get unsorted list')">
create new list after pasting in csv file</a>
<a href="javascript:msns20170225sa1747('sort to list')">
paste in three record urls and a list name like this: url,url,url,list name to sort this stock to a list</a>
<div id="msns20170225sa1747unsortedlist"></div>
<div id="msns20170225sa1747sortedlist"></div>
<div id="msns20170225sa1747listname"></div>
<div id="msns20170225sa1747currentposition"></div>
function msns20170225sa1747(request)
// from thinking about the sorter
function msns20170225sa1747(request) {
if (request === "sort to list") {sorttolist()}
if (request === "get new list") {getnewlist()}
if (request === "add history") {addhistory()}
function next(listname) {}
a record is created when we sort a stock to a category
stocks can be sorted to record categories
i mean, the unsorted list ...
let's see ... !
we might input a new list
some of the stocks on that list might already have been sorted to categories
how would we find out?
function hasthisbeensortedq() {}
if (hasthisbeensortedq() === "yes") {}
function nextunsortedstock() {}
nextunsortedstock() gets called when we're in sorting mode
meaning, when we're sorting a new list ... into the database
a stock gets sorted to a list when it becomes of interest
all stocks are of interest
ah ... an insight
sorting a stock into the system sets up an observation page
if the observation is of the buy type
at some point we will want to move it into a record category
when we do that we also split it into a new track or buy category
i'm not sure that's quite right
if a stock is in a bought category
at some point it will either move up or down ... or sideways
and we might want to record an additional observation
function addobservation() {}
so a single record could eventually contain multiple observations
surrounding the record chart are
a next / previous button set ... the next or previous observation for that record
a monthly / weekly / daily button set
which works for each observation
a next / previous set which goes to the next observation on this list
if (request === "next observation") {}
if (request === "previous observation {}
if (request === "monthly observation") {}
if (request === "weekly observation") {}
if (request === "daily observation") {}
if (request === "hourly observation") {}
if (request === "fifteen minute observation") {}
if (request === "next stock") {}
if (request === "previous stock") {}
if Irequest === "new category") {}
if (request === "new list") {}
if (request === "return to new list") {}
if (request === "save new list") {}
if (request === "save the database") {}
if (request === "create new list") {}
function msns20170225sa1747(request) {
if (request === "sort to list") {sorttolist()}
if (request === "get new list") {getnewlist()}
if (request === "add history") {addhistory()}
function next(listname) {}
a record is created when we sort a stock to a category
stocks can be sorted to record categories
i mean, the unsorted list ...
let's see ... !
we might input a new list
some of the stocks on that list might already have been sorted to categories
how would we find out?
function hasthisbeensortedq() {}
if (hasthisbeensortedq() === "yes") {}
function nextunsortedstock() {}
nextunsortedstock() gets called when we're in sorting mode
meaning, when we're sorting a new list ... into the database
a stock gets sorted to a list when it becomes of interest
all stocks are of interest
ah ... an insight
sorting a stock into the system sets up an observation page
if the observation is of the buy type
at some point we will want to move it into a record category
when we do that we also split it into a new track or buy category
i'm not sure that's quite right
if a stock is in a bought category
at some point it will either move up or down ... or sideways
and we might want to record an additional observation
function addobservation() {}
so a single record could eventually contain multiple observations
surrounding the record chart are
a next / previous button set ... the next or previous observation for that record
a monthly / weekly / daily button set
which works for each observation
a next / previous set which goes to the next observation on this list
if (request === "next observation") {}
if (request === "previous observation {}
if (request === "monthly observation") {}
if (request === "weekly observation") {}
if (request === "daily observation") {}
if (request === "hourly observation") {}
if (request === "fifteen minute observation") {}
if (request === "next stock") {}
if (request === "previous stock") {}
if Irequest === "new category") {}
if (request === "new list") {}
if (request === "return to new list") {}
if (request === "save new list") {}
if (request === "save the database") {}
if (request === "create new list") {}
Friday, February 24, 2017
function show(request) {}
function show(request) {
/*
request come in these flavors: owned, actually owned, and not owned
each of these comes in three flavors: line, spade, and shoulder ... for now
so
show requests look like this:
owned lines
owned spades
owned shoulders
actually owned lines
actually owned spades
actually owned shoulders
not owned lines
not owned spades
not owned shoulders
thus
*/
var requestarray = request.split(" ")
var requesettype = requestarray[0]
var requestedlist = requestarray[requestarray.length - 1]
if (requesttype === "owned") {showowned(requestedlist)}
if (requesttype === "actually") {showactuallyowned(requestedlist)}
if (requesttype === "not") {shownotowned(requestedlist)}
}
/*
request come in these flavors: owned, actually owned, and not owned
each of these comes in three flavors: line, spade, and shoulder ... for now
so
show requests look like this:
owned lines
owned spades
owned shoulders
actually owned lines
actually owned spades
actually owned shoulders
not owned lines
not owned spades
not owned shoulders
thus
*/
var requestarray = request.split(" ")
var requesettype = requestarray[0]
var requestedlist = requestarray[requestarray.length - 1]
if (requesttype === "owned") {showowned(requestedlist)}
if (requesttype === "actually") {showactuallyowned(requestedlist)}
if (requesttype === "not") {shownotowned(requestedlist)}
}
function mmns20170224f1351(request) {}
function mmns20170224f1351(request) {
if (request === "show owned lines") {show('owned lines')}
if (request === "show owned spades") {show('owned spades')}
if (request === "show owned shoulders") {show('owned shoulders')}
if (request === "show not owned lines") {show('owned lines')}
if (request === "show not owned spades") {show('owned spades')}
if (request === "show not owned shoulders") {show('owned shoulders')}
if (request === "show the charts") {showthecharts()}
if (request === "show next record") {shownextrecord()}
if (request === "show previous record") "showpreviousrecord()}
I think I'm seeing room for development in here.
if (request.split(" ")[0] === "show") {
show(request.split(" ").slice(1).join(" "))}
}
if (request === "show owned lines") {show('owned lines')}
if (request === "show owned spades") {show('owned spades')}
if (request === "show owned shoulders") {show('owned shoulders')}
if (request === "show not owned lines") {show('owned lines')}
if (request === "show not owned spades") {show('owned spades')}
if (request === "show not owned shoulders") {show('owned shoulders')}
if (request === "show the charts") {showthecharts()}
if (request === "show next record") {shownextrecord()}
if (request === "show previous record") "showpreviousrecord()}
I think I'm seeing room for development in here.
if (request.split(" ")[0] === "show") {
show(request.split(" ").slice(1).join(" "))}
}
Thursday, February 23, 2017
some groups
thinking about ... the sorter tu201702281122new
developing software to support a process
lines. spades. shoulders.
own, metaphorically speaking. don't own.
<span id="mmns20170224f1351ownedlines"></span>
<a href="javascript:mmns20170224f1351('show owned lines')"></a>
<a href="javascript:mmns20170224f1351('hide the charts')"></a>
<img src="" id="mmns20170224f1351record" />
<img src="" id="mmns20170224f1351updater />
<a href="" id="mmns20170224f1351flink" target="_blank">f</a>
<a href="" id="mmns20170224f1351mlink" target="_blank">m</a>
I am going to do something very strange, here, and turn the show owned lines link and the hide the charts link into links right here in Blogger's compose mode. Well, hide the charts may need to move to some other location, but this way some of these links can be selected right from here, in this blog's text.
To do this, I will copy from here the whole show owned lines link, and, in HTML Mode in Blogger Edit, I'll paste that into ... no ... no ... it's not even that. In Compose Mode I can turn the above link code into a link, and supply the href for that, which is the javascript call, all using the link dialog.
Other parts of the code in this text I can copy, from here, and paste into HTML Mode, which will add them as parts of the HTML (while leaving this text here as it is).
I wonder if maybe I ought to break out the JavaScript into a separate page. I'm thinking it might be an idea.
function mmns20170224f1351(request) {}
developing software to support a process
lines. spades. shoulders.
own, metaphorically speaking. don't own.
<span id="mmns20170224f1351ownedlines"></span>
<a href="javascript:mmns20170224f1351('show owned lines')"></a>
<a href="javascript:mmns20170224f1351('hide the charts')"></a>
<img src="" id="mmns20170224f1351record" />
<img src="" id="mmns20170224f1351updater />
<a href="" id="mmns20170224f1351flink" target="_blank">f</a>
<a href="" id="mmns20170224f1351mlink" target="_blank">m</a>
I am going to do something very strange, here, and turn the show owned lines link and the hide the charts link into links right here in Blogger's compose mode. Well, hide the charts may need to move to some other location, but this way some of these links can be selected right from here, in this blog's text.
To do this, I will copy from here the whole show owned lines link, and, in HTML Mode in Blogger Edit, I'll paste that into ... no ... no ... it's not even that. In Compose Mode I can turn the above link code into a link, and supply the href for that, which is the javascript call, all using the link dialog.
Other parts of the code in this text I can copy, from here, and paste into HTML Mode, which will add them as parts of the HTML (while leaving this text here as it is).
I wonder if maybe I ought to break out the JavaScript into a separate page. I'm thinking it might be an idea.
function mmns20170224f1351(request) {}
Wednesday, February 22, 2017
lnth
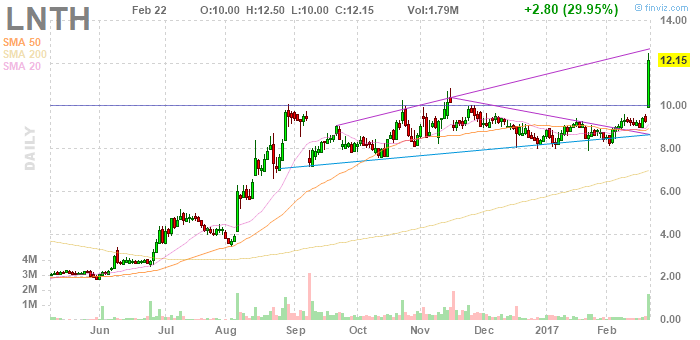
today's charts are all kind of whacked except maybe $LNTH
— Tom Sunderland (@atnbg) Feb. 22 at 11:17 PM
Tuesday, February 21, 2017
rearing up 2
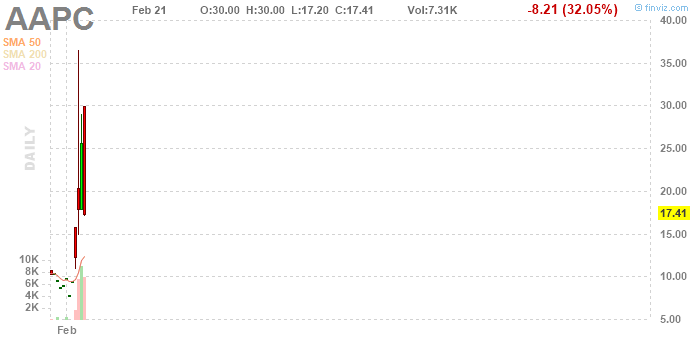
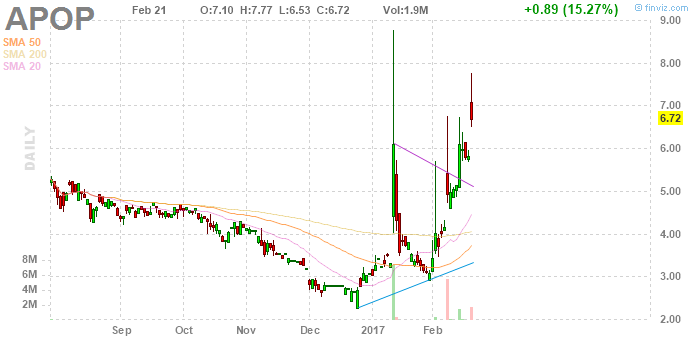
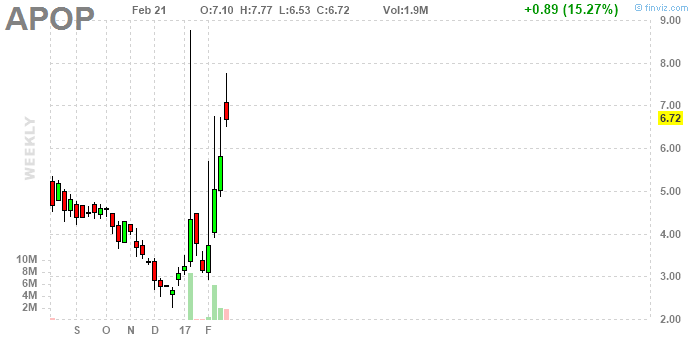
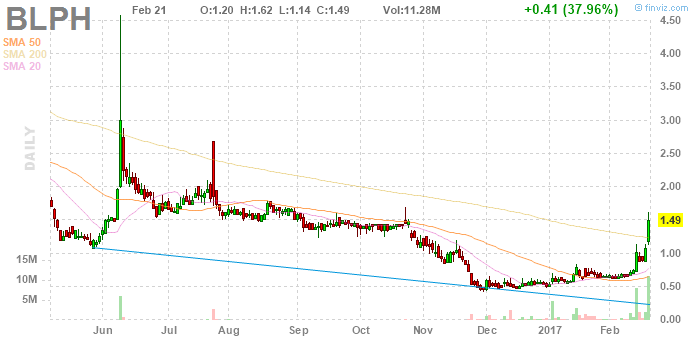
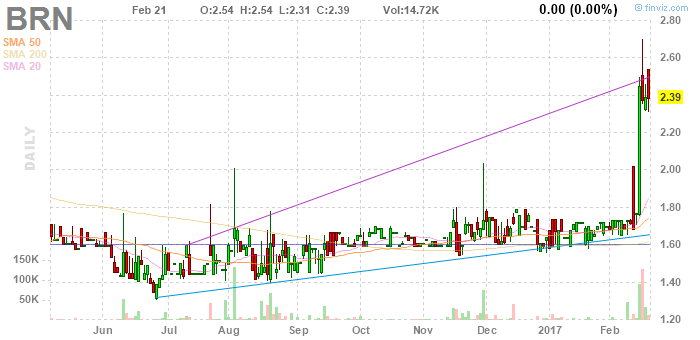


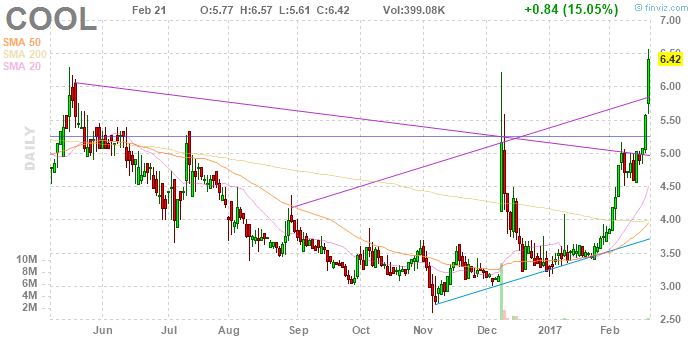
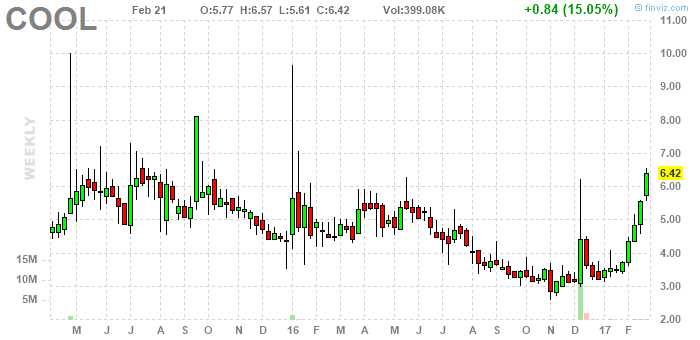
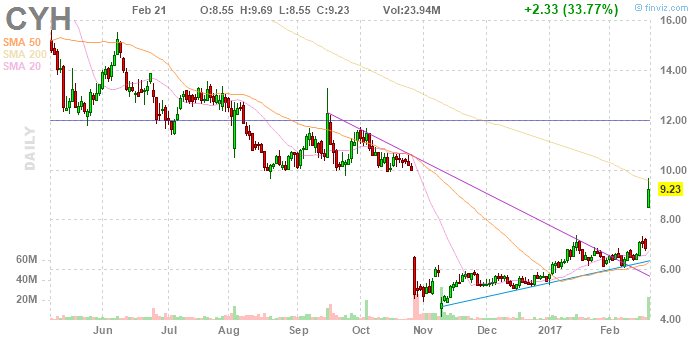
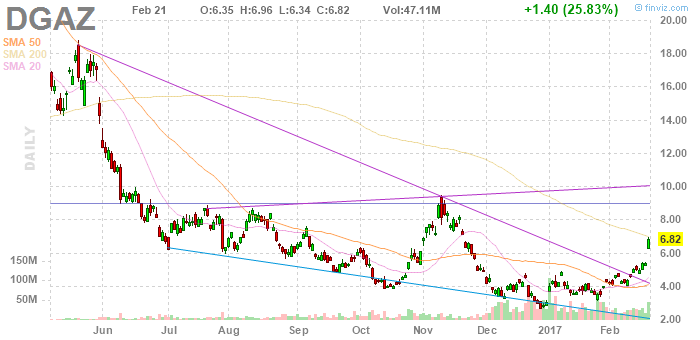
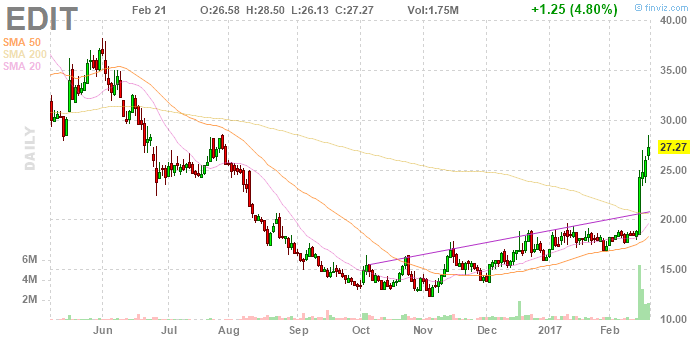
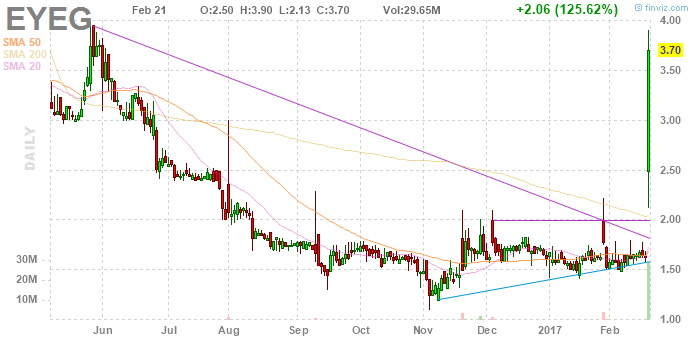
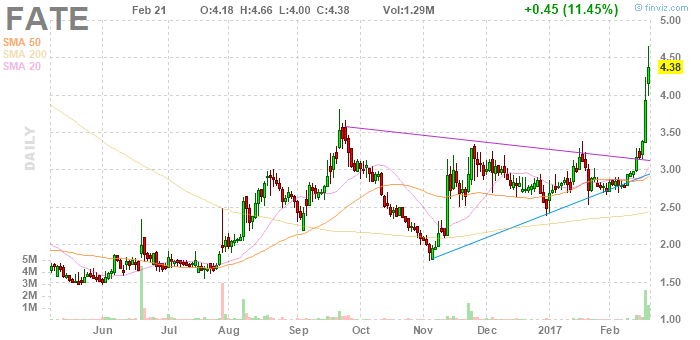
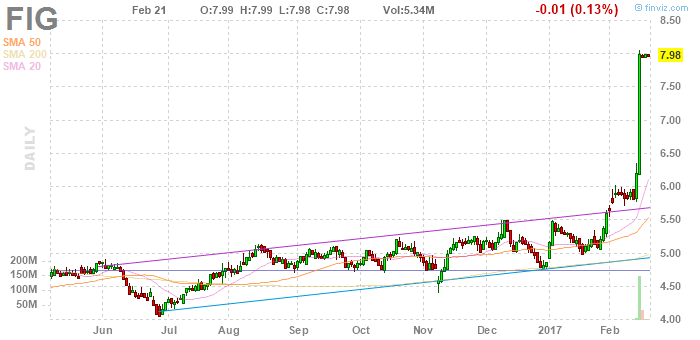
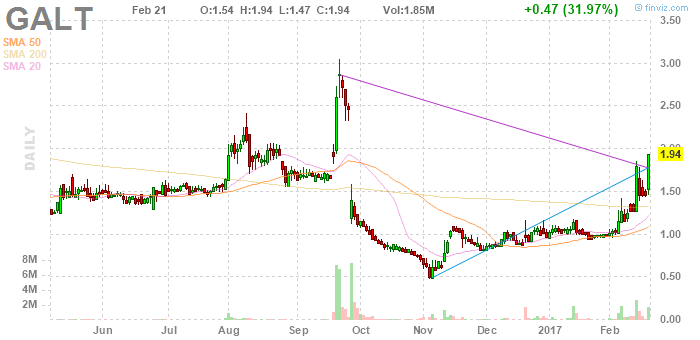
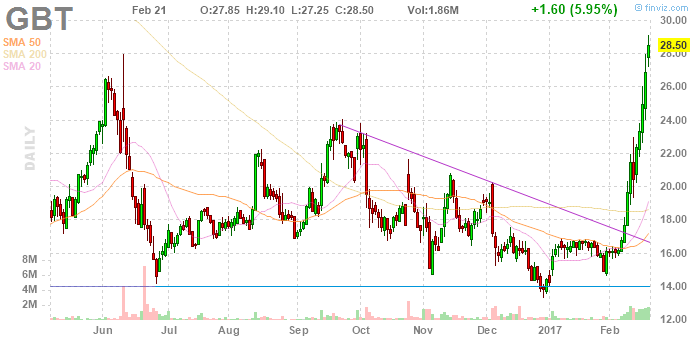
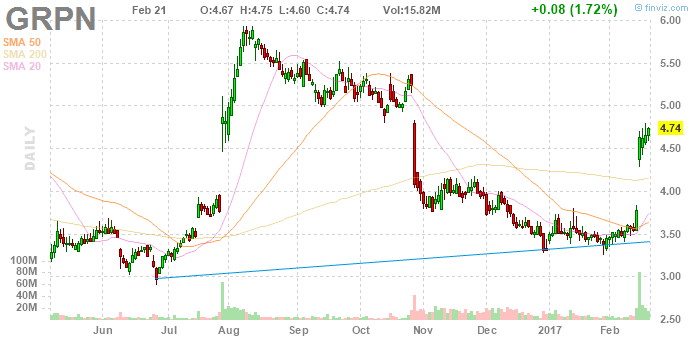
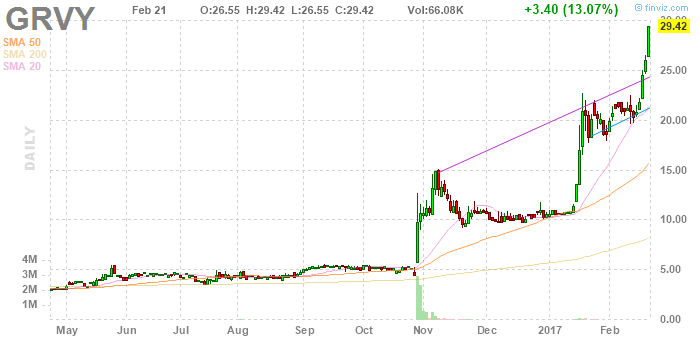
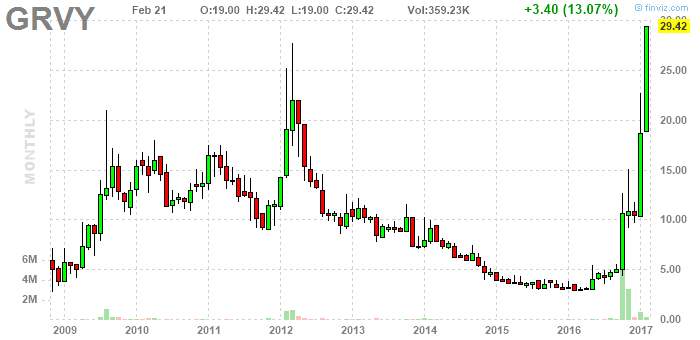
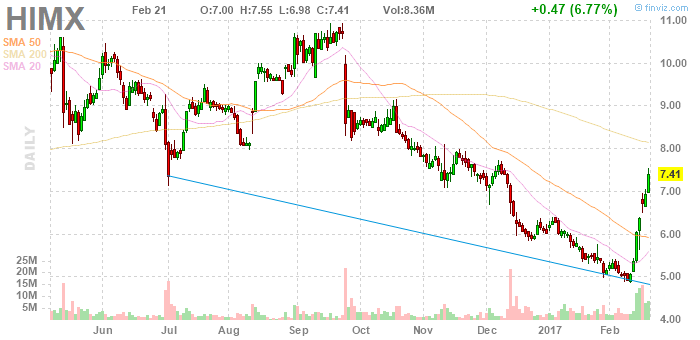
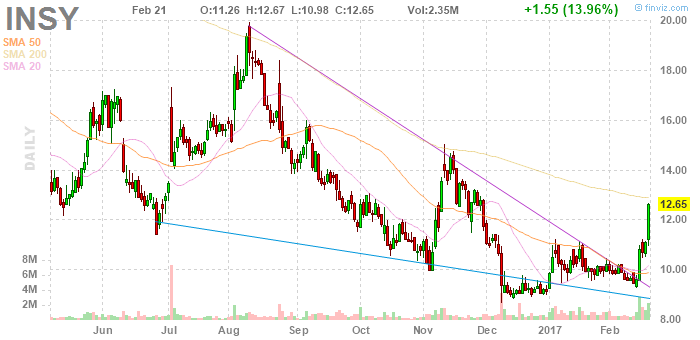
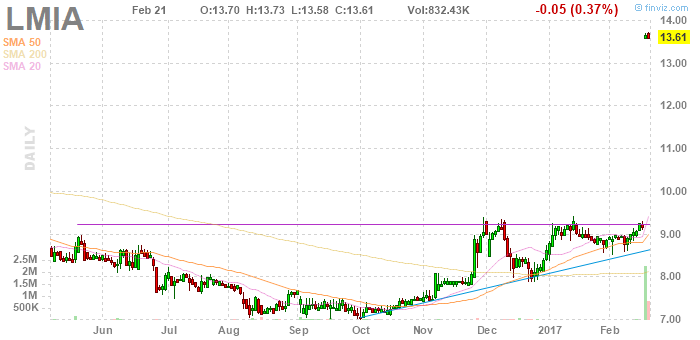
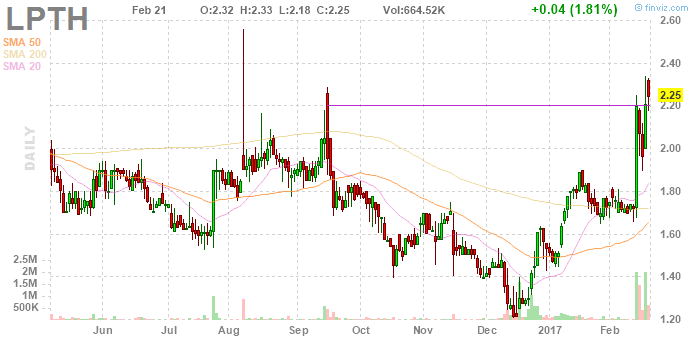
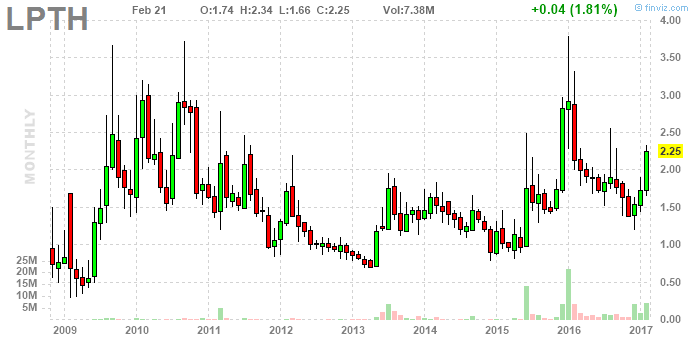
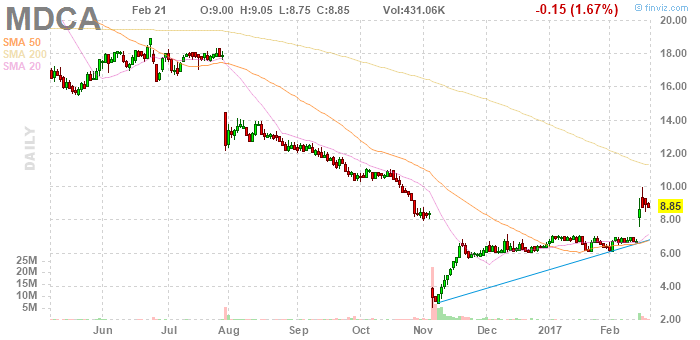
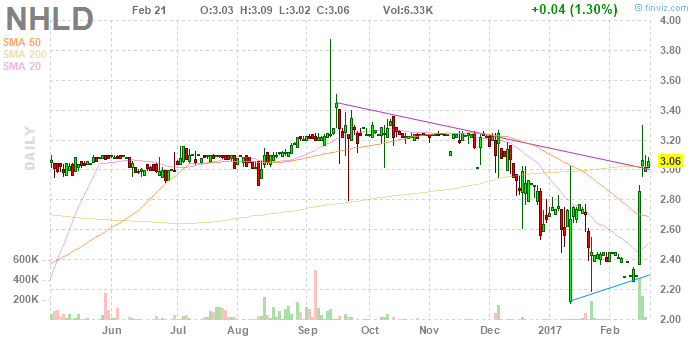
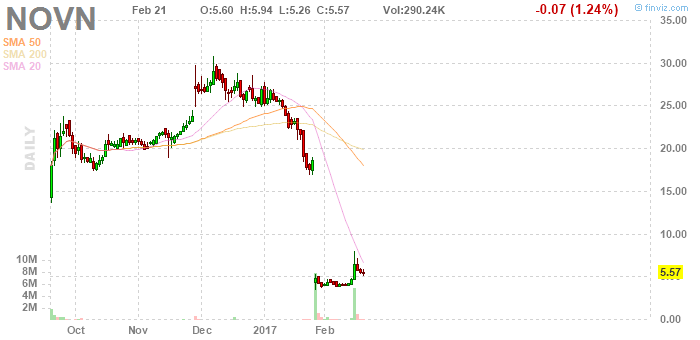
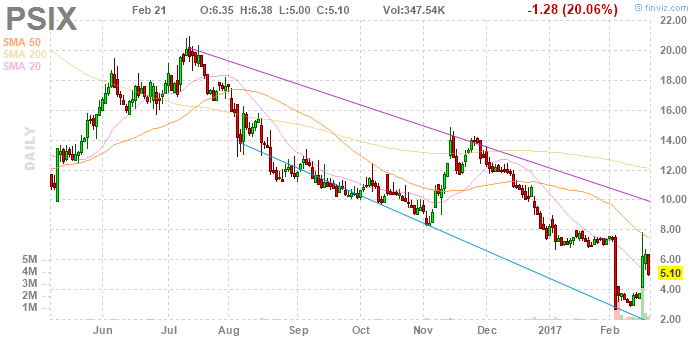
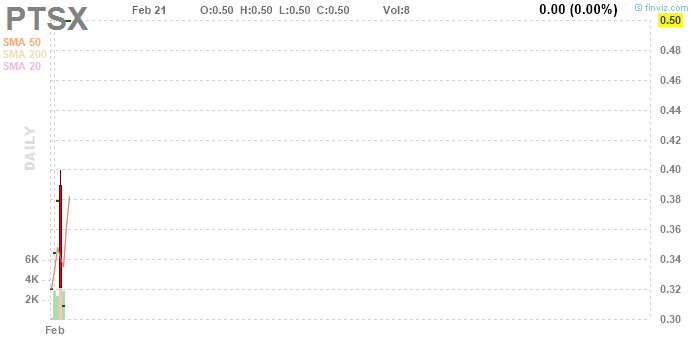
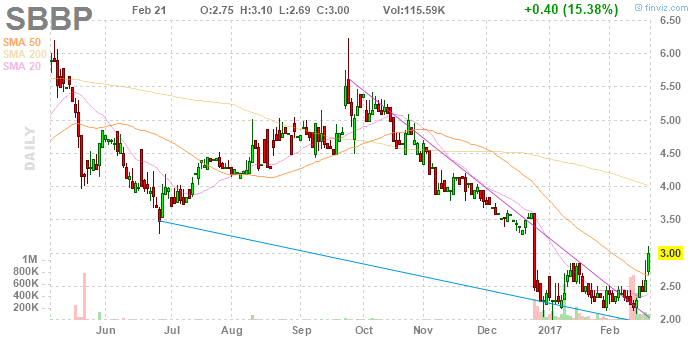
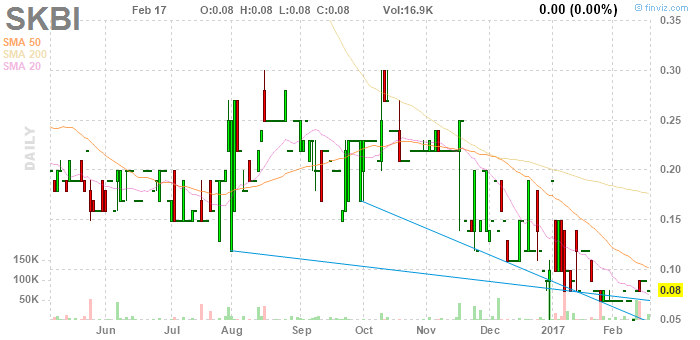
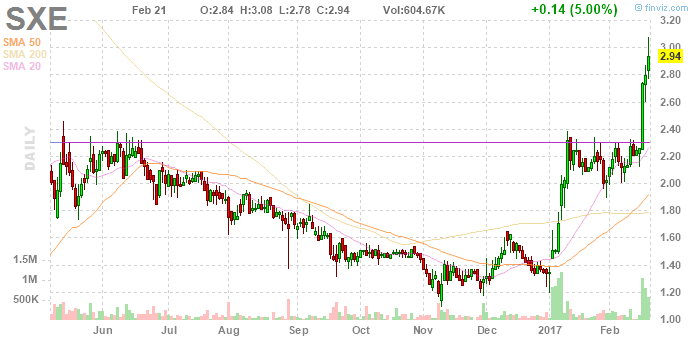
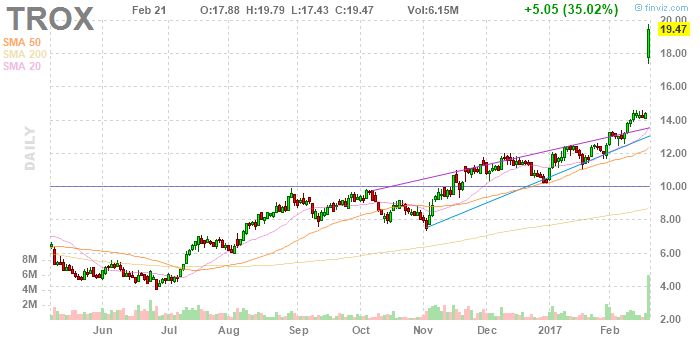
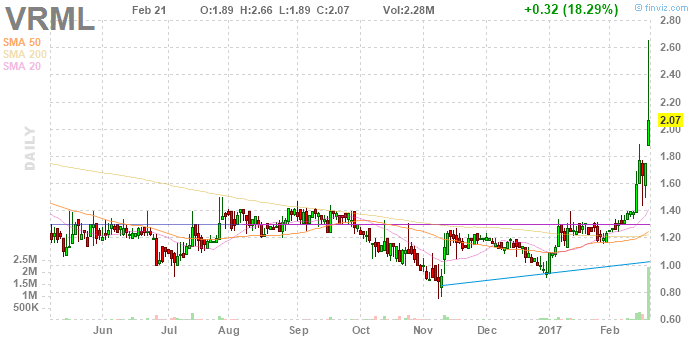
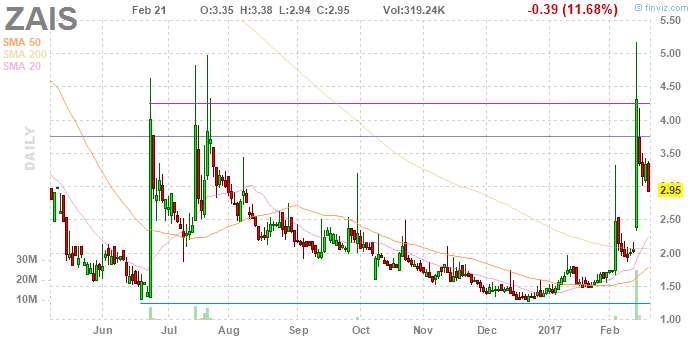
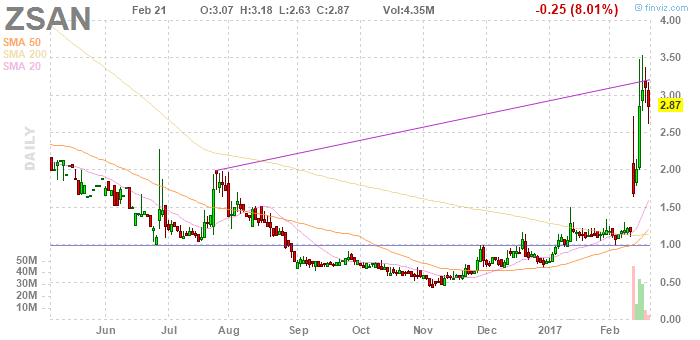
Subscribe to:
Posts (Atom)